NumPyとNLCPyの間の相互運用性
NLCPyは、NumPyのAPIのサブセットを提供します。 ただし、NLCPyのndarray(nlcpy.ndarray
)は __array__ メソッドを実装しています。これにより、NLCPyのndarrayをNumPyの関数に渡すことができます。
例1:
>>> import nlcpy
>>> import numpy
>>>
>>> x = nlcpy.arange(3)
>>> y = nlcpy.arange(3)
>>> numpy.meshgrid(x, y)
[array([[0, 1, 2],
[0, 1, 2],
[0, 1, 2]]), array([[0, 0, 0],
[1, 1, 1],
[2, 2, 2]])]
numpy.meshgrid はNumPyの関数であるのに対し、 x
と y
はどちらもNLCPyのndarrayであることに注意してください。
注釈
NumPyの関数はx86ノード(VH)で実行されます。 一方、ほとんどのNLCPyの関数は、自動的にndarrayをVector Engine(VE)にオフロードしてから、VEで実行します。
numpy.ndarray
を使用する他のパッケージの関数に渡すこともできます。例2(Matplotlib):
>>> import nlcpy as vp
>>> import matplotlib.pylab as plt
>>>
>>> x = vp.linspace(-vp.pi, vp.pi, 201)
>>> type(x)
<class 'nlcpy.core.core.ndarray'>
>>>
>>> plt.plot(x, vp.sin(x))
...
>>> plt.xlabel('Angle [rad]')
...
>>> plt.ylabel('sin(x)')
...
>>> plt.axis('tight')
...
>>> plt.show()
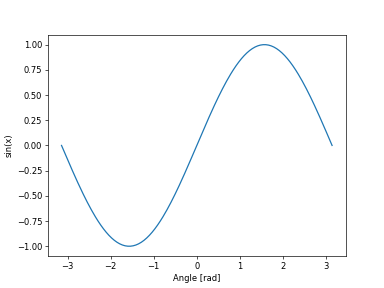
例3(Pandas):
>>> import nlcpy as vp
>>> import pandas as pd
>>>
>>> x = vp.random.rand(3,3)
>>> pd.DataFrame(x, index=list('abc'), columns=list('ABC'))
A B C
a 0.6575677252840251 0.7966675218194723 0.5927528077736497
b 0.1310200293082744 0.0033949704375118017 0.4242931657936424
c 0.343795241555199 0.88619629223831 0.9364728704094887
nlcpy.ndarray
と numpy.ndarray
の間で変換する場合は、 nlcpy.asarray()
と numpy.asarray() を使用する必要があります。
例4では、 nlcpy.asarray()
がNumPy ndarrayをVHからVEに転送し、ndarrayはNLCPyのndarrayとして表されます。 逆に、 numpy.asarray() はndarrayをVEからVHに転送します。
例4 :
>>> x = numpy.arange(5)
>>> type(x)
<class 'numpy.ndarray'>
>>>
>>> x = nlcpy.asarray(x) # converts from numpy.ndarray to nlcpy.core.core.ndarray
>>> type(x)
<class 'nlcpy.core.core.ndarray'>
>>>
>>> x = numpy.asarray(x) # converts from nlcpy.core.core.ndarray to numpy.ndarray
>>> type(x)
<class 'numpy.ndarray'>
>>>
>>> x
array([0, 1, 2, 3, 4])
さらに、 nlcpy.ndarray.get()
は、VEからVHにデータを転送したNumPyのndarrayを返します。
例5 :
>>> x = nlcpy.arange(5)
>>> type(x)
<class 'nlcpy.core.core.ndarray'>
>>>
>>> y = x.get() # converts from nlcpy.core.core.ndarray to numpy.ndarray.
>>> type(y)
<class 'numpy.ndarray'>
>>>
>>> y
array([0, 1, 2, 3, 4])
注釈
NLCPyの関数の引数に nlcpy.ndarray
と numpy.ndarray
の両方を渡すと、関数は結果を nlcpy.ndarray
として返します。 逆に、NumPyの関数は結果を numpy.ndarray
として返します。
>>> import numpy, nlcpy
>>> x = nlcpy.arange(10)
>>> y = numpy.arange(10)
>>>
>>> type(x+y)
<class 'nlcpy.core.core.ndarray'> # ndarray of nlcpy
>>>
>>> type(nlcpy.add(x,y))
<class 'nlcpy.core.core.ndarray'> # ndarray of nlcpy
>>>
>>> type(numpy.add(x,y))
<class 'numpy.ndarray'> # ndarray of numpy
NumPyへの自動置換
v2.2.0から、NLCPyは未実装の関数やメソッドのほとんどを自動的にNumPyの関数やメソッドに置換します。
v2.2.0以前:
>>> import nlcpy >>> nlcpy.nancumsum(nlcpy.array(1)) Traceback (most recent call last): File "<stdin>", line 1, in <module> AttributeError: module 'nlcpy' has no attribute 'nancumsum'
v2.2.0から:
>>> import nlcpy >>> nlcpy.nancumsum(nlcpy.array(1)) array([1]) # 1. transfer argument value to VH # 2. execute NumPy's function # 3. transfer result value to VE
もしこの機能を制限したい場合は、環境変数 VE_NLCPY_ENABLE_NUMPY_WRAP
に NO
もしくは no
を設定してください。デフォルトは YES
です。
$ VE_NLCPY_ENABLE_NUMPY_WRAP=NO python >>> import nlcpy >>> nlcpy.nancumsum(nlcpy.array(1)) ... NotImplementedError: nancumsum is not implemented yet.
注釈
Python3.6を利用しているとき、この機能はモジュールレベルの関数 ( nlcpy.xxx
, nlcpy.linalg.xxx
, nlcpy.random.xxx
など) に対して動作しません。